Introduction
Managing over a thousand news websites presents unique challenges in maintaining a consistent design and efficiently sharing content to social media.
This report proposes a centralized system that leverages WordPress Multisite for a single reusable theme and a custom plugin to automate social media publishing across multiple platforms.
The goal is to ensure each brand site can instantly share newly published articles (with featured images, excerpts, and hashtags) to all relevant social channels, via a unified dashboard.
We emphasize a scalable architecture (to handle 1000+ sites), performance optimizations for rapid auto-posting, and robust security for managing numerous social media account credentials.
This report is an actual case study of how we developed our own in-house social publishing platform for my network of 1000+ and growing global news brands.
Requirements Overview
The system must fulfill the following key requirements (grouped by functionality):
Unified Theme & Site Management:
Develop a single WordPress theme (template) that is reused across all brand sites for a consistent look and easier maintenance.
Support deployment to 1000+ WordPress sites, preferably via a centralized mechanism (to avoid managing each separately).
Centralized Social Publishing:
From-Scratch Implementation:
The solution cannot rely on pre-existing plugins or services; we must build a custom WordPress plugin for automation and provide all necessary code.
The system should allow connecting social accounts per brand through a UI, storing credentials (API keys/tokens) securely, and managing each brand’s accounts independently.
Provide a step-by-step deployment guide for installing the theme and plugin on each site (or network) and instructions to onboard each brand into the social publishing platform.
Performance, Scalability, Security:
Design for minimal delay in publishing to 10+ networks per post, without slowing down the writer’s publishing workflow. Possibly utilize background processing or queuing to handle API calls.
Ensure the architecture can scale to support thousands of sites and social accounts (e.g. efficient database structure, caching, load balancing as needed).
Secure handling of credentials and API interactions (e.g. OAuth flows, encrypted storage, permission scopes) to protect sensitive data and prevent unauthorized access.
These requirements will be addressed through two main components: a WordPress Multisite network with a shared theme for all sites, and a custom “Social Publisher” plugin that provides the centralized dashboard and social automation features.
System Architecture
WordPress Multisite is the foundation of the system, enabling all brand sites to reside in a single WordPress installation. Multisite allows running multiple websites from one dashboard and codebase, while giving each site a unique domain and data set.
In this architecture:
Single Code Base, Multiple Domains: We configure WordPress Multisite such that each news brand is a site on the network with its own domain (e.g. brand1.com
, brand2.com
, …, up to brand1000.com
).
WordPress Multisite natively supports mapping each site to a separate domain, so each brand retains its identity and URL. All sites share the same theme and plugins, making updates and new features instantly available to all.
Centralized Management: The network is administered by a Super Admin who can install the theme/plugin once for all sites. Each brand’s team can be given admin access to their own site (their “workspace”) to publish content and manage social accounts, while the Super Admin oversees the entire network.
This setup drastically simplifies maintenance – e.g., updating the theme or plugin happens in one place for 1000+ sites.
Custom Social Publishing Plugin: A custom plugin (installed network-wide) provides a Central Social Media Publisher Dashboard.
This dashboard is accessible in each site’s WP Admin for brand-specific configuration, and can also have a network-level view for the Super Admin if needed.
The plugin handles connecting to all required social media APIs and auto-posting content when a new article is published. It essentially acts as a bridge between each WordPress site and multiple social networks.
Figure: High-Level Architecture – The diagram below illustrates the architecture. Each brand site in the WordPress Multisite network uses the shared theme and interacts with the central social publishing plugin.
When an editor publishes a post, the plugin immediately distributes the post to all configured social platforms for that brand. All social account credentials and settings are managed through the plugin’s dashboard, keeping things centralized for admins while isolating each brand’s data.
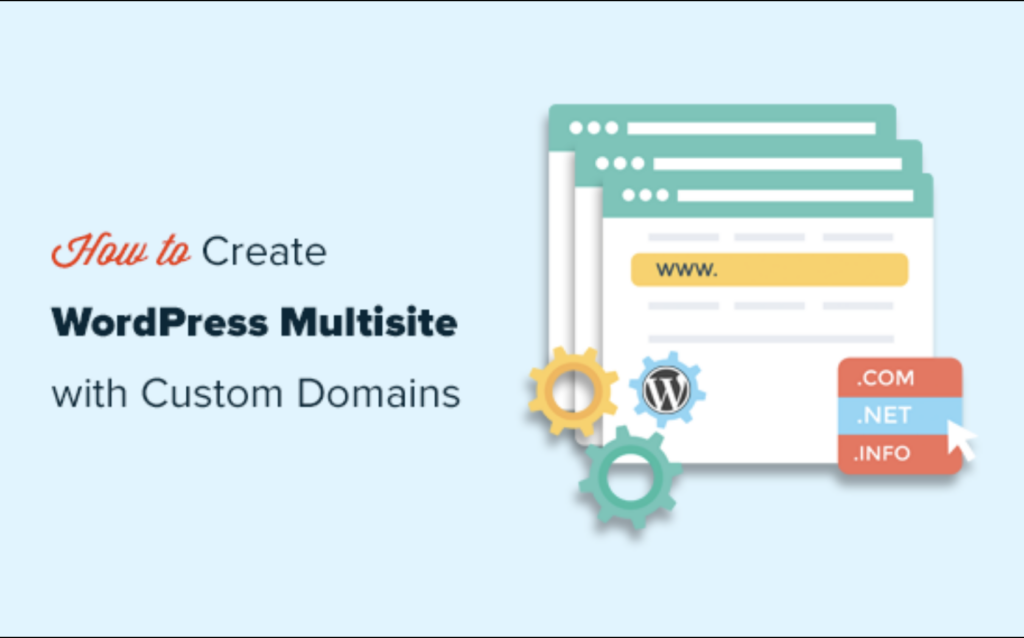
WordPress Multisite network with multiple brand sites on one installation (each with a custom domain). This setup allows centralized management of the theme and plugins across all sites.
(The above illustration demonstrates how a single WordPress Multisite installation can host multiple sites with distinct domains, simplifying updates and maintenance by sharing themes/plugins.)
In this architecture, performance and scalability are addressed by the nature of Multisite and our plugin design: WordPress Multisite is highly scalable, known to handle thousands of sites on a single instance.
Each site has its own database tables, reducing query load contention across sites. With proper caching and a robust server setup, even a network of this size can run efficiently on one codebase.
We will use object caching and page caching to ensure that even with 1000 sites, the load on the database and server is minimized for normal visitors. The social posting operations will be handled asynchronously to avoid impacting page load times (details in the plugin design section).
Next, we detail the two primary components of the system: the reusable WordPress theme for all news sites, and the Social Publisher plugin that automates multi-platform content sharing.
Reusable WordPress Theme for All News Sites
We create a custom WordPress theme that will be used by all 1000+ news sites. Having a single theme ensures consistent branding (if desired) and functionality across sites, and simplifies rolling out improvements. Key characteristics of the theme include:
Modern, Responsive Design: The theme uses a clean, newspaper-style layout that is mobile-friendly. It can be customized (via WordPress Customizer options) with each brand’s logo, colors, and typography, so that even though the code is the same, each site can maintain its unique look and feel.
News-Oriented Features: It supports common news site needs – e.g. multiple category sections, featured post highlights, author bio sections, etc.
The theme will leverage WordPress features like custom menus (for navigation), widgets (for sidebars/footer), and featured images for thumbnails. It will also support excerpts so that on listing pages (home, category archives) each post shows a snippet.
Performance Optimizations: The theme will be lightweight. We avoid bloated page builders or unnecessary external calls. We enable page caching and use lazy loading for images to ensure fast page loads.
Where possible, the theme will defer heavy scripts and use efficient queries (e.g., using WordPress’s $wpdb
or WP_Query
properly to fetch posts, and caching results). Since all sites share this theme, any performance improvement benefits the entire network.
Security Best Practices: The theme’s templates properly escape output (using esc_html
, esc_url
, etc.) to prevent XSS, and sanitize inputs for any theme settings. It doesn’t expose any sensitive data. This is crucial as it will be deployed widely.
Below is the complete code for the core theme files, which demonstrates a basic but scalable news theme structure:
style.css
– Theme meta info and basic styles:
cssCopy/*!
Theme Name: Central News Theme
Theme URI: https://example.com/central-news-theme
Author: [Your Name/Company]
Description: A reusable theme for multi-brand news sites, with a clean responsive design.
Version: 1.0
License: GNU General Public License v2 or later
Text Domain: central-news
*/
body {
font-family: Arial, sans-serif;
background-color: #fff;
color: #333;
margin: 0;
padding: 0;
}
.header, .footer { background: #000; color: #fff; padding: 20px; text-align: center; }
.nav-menu { margin: 10px 0; }
.post-excerpt img { max-width: 100%; height: auto; }
...
functions.php
– Set up theme supports and dynamic features:
phpCopy<?php
// Enable featured images, dynamic title tags, menus, etc.
function centralnews_theme_setup() {
add_theme_support('post-thumbnails'); // Featured images
add_theme_support('title-tag'); // Document title tag control
add_theme_support('html5', ['search-form', 'gallery', 'caption']);
register_nav_menu('main-menu', 'Main Navigation Menu');
}
add_action('after_setup_theme', 'centralnews_theme_setup');
// Enqueue theme stylesheet (and optionally scripts)
function centralnews_enqueue_assets() {
wp_enqueue_style('centralnews-style', get_stylesheet_uri(), [], '1.0');
}
add_action('wp_enqueue_scripts', 'centralnews_enqueue_assets');
?>
header.php
– Common header for all pages (branding and navigation):
phpCopy<!DOCTYPE html>
<html <?php language_attributes(); ?>>
<head>
<meta charset="<?php bloginfo('charset'); ?>" />
<meta name="viewport" content="width=device-width, initial-scale=1" />
<?php wp_head(); // WordPress head hook for scripts, styles, meta ?>
</head>
<body <?php body_class(); ?>>
<header class="header">
<div class="site-branding">
<?php
// Display site title or logo
if ( function_exists('the_custom_logo') && has_custom_logo() ) {
the_custom_logo();
} else {
echo '<h1>'. get_bloginfo('name') .'</h1>';
}
?>
</div>
<nav class="nav-menu">
<?php wp_nav_menu(['theme_location' => 'main-menu']); ?>
</nav>
</header>
<main class="content">
footer.php
– Common footer:
phpCopy </main> <!-- end .content -->
<footer class="footer">
<p>© <?php echo date('Y'); ?> <?php bloginfo('name'); ?>. All rights reserved.</p>
</footer>
<?php wp_footer(); // WordPress footer hook ?>
</body>
</html>
index.php
– Main template for listing posts (home or archive pages):
phpCopy<?php get_header(); ?>
<div class="post-list">
<?php
if (have_posts()):
while (have_posts()): the_post(); ?>
<article id="post-<?php the_ID(); ?>" <?php post_class('post-excerpt'); ?>>
<?php if (has_post_thumbnail()): ?>
<a href="<?php the_permalink(); ?>"><?php the_post_thumbnail('medium'); ?></a>
<?php endif; ?>
<h2 class="post-title"><a href="<?php the_permalink(); ?>"><?php the_title(); ?></a></h2>
<div class="post-meta">
<span class="post-date"><?php the_time( get_option('date_format') ); ?></span> |
<span class="post-author"><?php the_author(); ?></span>
</div>
<div class="post-summary">
<?php the_excerpt(); // Display excerpt of the content ?>
</div>
<a class="read-more" href="<?php the_permalink(); ?>">Read more »</a>
</article>
<?php endwhile;
// Pagination links if many posts
the_posts_pagination();
else:
echo '<p>No posts found.</p>';
endif;
?>
</div>
<?php get_footer(); ?>
single.php
– Template for individual news articles:
phpCopy<?php get_header(); ?>
<?php if(have_posts()): the_post(); ?>
<article id="post-<?php the_ID(); ?>" <?php post_class('post-single'); ?>>
<h1 class="post-title"><?php the_title(); ?></h1>
<div class="post-meta">
<span class="post-date"><?php the_time( get_option('date_format') ); ?></span> |
<span class="post-author">By <?php the_author(); ?></span>
</div>
<?php if(has_post_thumbnail()): ?>
<div class="post-featured-image"><?php the_post_thumbnail('large'); ?></div>
<?php endif; ?>
<div class="post-content">
<?php the_content(); ?>
</div>
<div class="post-tags">
<?php the_tags('<span class="tag">#','</span> <span class="tag">#','</span>'); ?>
</div>
</article>
<?php endif; ?>
<?php get_footer(); ?>
(Code comments: The theme displays posts with featured images and excerpts on listing pages, and full content on single pages. It uses built-in WP functions to output title, date, author, etc.
Each site can set its own title, logo, menu, and manage content normally. The HTML/CSS can be expanded for a more complex layout as needed. The above is a simplified baseline for clarity.)
Theme Deployment: Once this theme is placed in the /wp-content/themes/
directory of the network, the Super Admin can Network Enable it, making it available to all sites.
Each brand site can then activate this theme. Because all sites use the same theme files, updates to the theme propagate network-wide.
This satisfies the requirement of a single reusable template across all brands, easing maintenance and ensuring uniform capabilities on every site.
Centralized Social Media Publishing Plugin
The core of the system is the Social Publisher plugin that handles automated sharing of posts to social media. This plugin is custom-built to meet our specific needs (no existing automation plugin is used). Key responsibilities of the plugin include:
Account Management UI: Provide a secure interface (in WP Admin) for each brand to connect their social media accounts. This UI will appear as a “Social Publishing” section in each site’s dashboard.
Within it, brand admins can add or remove accounts for Facebook, Instagram, Twitter/X, etc., authorize the plugin to post on their behalf (via OAuth flows or API tokens), and set any platform-specific preferences (e.g. default hashtags, whether to include a link or not, etc.).
Each brand’s credentials are stored separately (e.g., in that site’s options table), so brands cannot see each other’s info. The plugin supports creating these separate workspaces automatically by virtue of the Multisite separation – each site’s settings pertain only to that site.
Auto-Publish Logic: The plugin hooks into WordPress post events. Specifically, it uses the publish_post
hook (and equivalents for custom post types if needed) to detect when a new article is published.
When triggered, it will immediately push that post’s content to all configured social channels for that site. The content sent includes the post title, an excerpt (or a truncated version of the post if no excerpt is set), a link back to the article on the site, the featured image, and hashtags (derived from the post’s tags or a predefined set).
This ensures the social posts are engaging and informative. The plugin ensures that as soon as an article goes live on the site, it is also live on all the brand’s social profiles, maximizing reach without manual effort.
Multi-Platform Support: We implement integrations for each required social network’s API. The following table outlines the platforms and how the plugin will publish to them, including content format and API method:
Table: Supported Social Platforms & Integration Details
Platform | API & Method | Content Shared | Notes |
---|---|---|---|
Facebook(Profiles & Pages) | Graph API (Facebook for Developers). Use the /me/feed endpoint for profiles (limited availability) or /PAGE-ID/feed for pages. | Post message (text), attached link to article (which generates a preview with image), or directly upload image with the post message. Hashtags included in message. | Profile posting via API is restricted – primarily pages are supported officially. The plugin will use a Facebook App with “manage_pages” and “publish_pages” permissions. Each brand authorizes their page. Featured image is either auto-previewed from link or explicitly attached for consistency. |
Instagram(Business) | Instagram Graph API (via Facebook). Use /IG-ACCOUNT-ID/media to publish. | Photo post with caption. The caption will contain the excerpt and hashtags. A shortened URL to the article can be included in caption text (not clickable, but for reference). | Requires an Instagram Business account linked to a Facebook Page and an Instagram Graph API token (with instagram_content_publish permission). The plugin will likely upload the featured image and caption. No clickable links in captions – we might include a phrase like “Link in bio” or rely on the profile bio link. |
Twitter/X(Free & Premium) | Twitter API v2 (or v1.1) via OAuth 1.0a user tokens. Use statuses/update (v1.1) or equivalent v2 endpoint for posting Tweet. | Tweet text (up to 280 chars for free API, more for premium accounts) including title or excerpt and link. The featured image can be uploaded via media API and attached to the Tweet, or omitted to let Twitter’s link preview use the site’s OpenGraph image. Hashtags appended to tweet text. | Twitter’s new API tiers have rate limits. Each brand will need a Twitter developer app or use a central app with user authentication. The plugin should handle OAuth for each account. We include 1-3 hashtags (Twitter best practice) from the post tags. If image is uploaded, the link still remains for click-through. |
LinkedIn(Profiles & Company Pages) | LinkedIn v2 Share API. Use /ugcPosts or /shares endpoint with OAuth2 token. | Post text (can include up to 1300 characters) including excerpt and link. The featured image URL can be included so LinkedIn fetches it for preview (or we attach via imageUrl in the API payload if allowed). Hashtags included inline in the text (LinkedIn supports hashtags). | LinkedIn requires separate permissions for posting to user profiles vs company pages (w_member_social and w_organization_social ). Each brand will authorize either/both. The plugin ensures to use the correct API path (profile vs organization). |
Threads(by Instagram) | API status: Currently no official public API for Threads (Meta’s platform) as of early 2025. We anticipate using an unofficial or custom integration. One approach is using the Threads private API or automation through the Instagram Graph if it becomes supported. | Post text (Threads are text-centric like Twitter). We would include a brief message with maybe a link (though Threads currently doesn’t hyperlink URLs) and hashtags. Images can be included as well if Threads supports it via API. | Because Threads is new, the plugin’s support for Threads may rely on third-party libraries or updates when Meta releases an API. For now, this could be an optional feature marked “beta”. Alternatively, if unavailable, an admin could be prompted to manually share until API exists. |
Bluesky(AT Protocol) | Bluesky has an API via the AT Protocol (App Passwords for authentication). Use the Bluesky API to create posts (content with facets for links and images). | Skeets (posts on Bluesky) with text content including link and hashtags. An image can be uploaded to Bluesky’s blob storage via API and attached to the post. | Each brand will provide their Bluesky credentials (or an app password) for the plugin to authenticate. The plugin will use the ATProto libraries to format posts. Bluesky being decentralized means each account’s server (usually bsky.social or custom) is used – our plugin will handle accordingly. |
Google My Business(Google Business Profile) | Google My Business API (Google Business Profile API). Use the accounts/{accountId}/locations/{locationId}/localPosts endpoint. | GMB posts require a title or summary, an image, and optionally a call-to-action with a link. The plugin will post an “Update” type post: a short summary of the article (100-300 words) and the featured image, with a CTA button linking to the news article. | Requires OAuth2 with Google (each brand needs to authorize their GMB location with scopes like https://www.googleapis.com/auth/business.manage ). The plugin will provide options to configure the CTA (e.g. “Read More” button). GMB posts have character limits and often expire after a week; the plugin might not need to re-post since it’s one-off news. |
Mastodon(Fediverse) | Mastodon API (ActivityPub/REST). Use /api/v1/statuses with an access token. | Toot content (up to 500 characters by default) including excerpt and link. We can attach the featured image by uploading it via /api/v1/media and then referencing the media ID in the status. Hashtags included as with Twitter. | Each brand must provide the Mastodon instance URL and a user token from that instance (since each could be on different servers). The plugin will store the instance base URL and token. Posting is similar to Twitter’s flow. We must abide by instance rules (e.g., some instances might have posting rate limits). |
Pinterest(Boards) | Pinterest Content API. Use /pins endpoint to create a Pin on a specified board. | The Pin will consist of the article’s featured image, a title (usually the post title), and a description (the excerpt + hashtags). The link of the Pin is set to the article URL, driving traffic back to the site. | Requires Pinterest developer app and OAuth token for each user. The brand admin will authorize Pinterest and select a board to post to. The plugin will upload the image to Pinterest and create the Pin. Hashtags in description are supported on Pinterest for searchability. |
TikTok(Business Accounts) | TikTok for Business API (Content Posting endpoint, if available). TikTok’s API allows scheduling posts via their business integration or TikTok’s Share to TikTok feature (videos only). | TikTok requires a video. This is challenging for text-based news. One approach is to create a short video clip (perhaps using the article’s image and title over music) as an automated process, or require the editor to provide a video snippet. If a video is available (attached to the post or generated), the plugin will upload it with a caption (including hashtags). | TikTok API integration requires business account and developer approval. If video content is not available, this integration might be optional. Alternatively, the plugin could skip TikTok unless a video field is provided in the post (e.g., a custom field for a short clip). This ensures only suitable content is shared. Hashtags are very important on TikTok, so the plugin will include relevant ones in the caption. |
YouTube Shorts | YouTube Data API (Videos). Use the /videos?part=snippet,status endpoint to upload a video file as a YouTube Short. | Like TikTok, Shorts requires video content (vertical format <60s). The plugin will take a video (if the news site produces a short video for the story, perhaps an autogenerated one or provided by editors) and upload it. The title and description fields on YouTube will be filled with the article title and a short excerpt, and hashtags (YouTube supports hashtags in descriptions). A link to the article can be included in the description. | Requires each brand’s YouTube channel OAuth (with scope youtube.upload ). If no video is present, the plugin will not post to YouTube for that article. (Alternatively, if the site has an associated video for the news, that can be used.) This may be an optional feature for brands that produce video content. |
(Table Notes:) All platforms will receive the content immediately after publication. The plugin will either call each API directly or use an SDK if available, handling authentication (OAuth flows for user authorization) and error checking.
Some networks have strict API quotas and posting rules; our implementation will include basic rate-limit handling (e.g., if an API call fails due to rate limits or network issues, the plugin will retry after a delay or log the failure for later).
The broad support is comparable to existing solutions like FS Poster, which supports auto-posting to 20+ networks. In fact, FS Poster’s capability to publish to “21 social networks and services from WordPress at the same time” proves that this multi-platform approach is feasible.
Our plugin is built with similar breadth in mind, covering all listed platforms (and it can be extended to new ones in the future if needed).
Notably, the plugin will allow customizing the message per platform to some extent. For example, maybe the brand wants a shorter message on Twitter than on Facebook, or different hashtags on LinkedIn than on Instagram.
The UI can let them enter a custom template or text for each platform on a per-post basis (this could be a meta box on the post editor to override the default message).
However, as a v1, we can start with a unified message format and later add per-platform tailoring. (The FS Poster plugin offers format customization per network, which is a useful feature to consider.)
Plugin Architecture & Data Storage
The Social Publisher plugin is structured to be efficient and secure:
It uses a modular design, where each social network integration is handled by a separate module or class. For instance, there might be classes like FacebookPublisher
, TwitterPublisher
, etc., each with a method publish(Post $post)
that knows how to format and send a post to that network. A central controller loops through all active integrations for the site when a post is published.
Storing Credentials: Each site’s social account tokens/keys are stored in the WordPress database. We leverage the options table or a custom table for this data. For security, we store refresh tokens or access tokens securely (encrypted using a secret key or using WordPress’s secret keys).
Alternatively, the plugin can rely on the OAuth tokens which are typically long random strings (safe to store), but we will not store any user passwords. Each token is scoped only to the ability to post on that platform, limiting potential damage.
Only administrators of that site (or the network super admin) can manage these credentials via the UI. The plugin will implement the WordPress Settings API with proper nonces and capability checks so that only authorized users can update the settings.
Central Dashboard vs Per-site Settings: In a Multisite environment, each site’s admin dashboard will show Social Publisher settings for that brand.
The Super Admin could be given a network-level overview page that lists all sites and which accounts are connected, etc., but direct management of accounts can be delegated to each brand’s admins.
This fulfills the “separate workspaces per brand” requirement: effectively, each site’s settings page is that brand’s workspace in the central system.
If we were not using Multisite, we would have had to implement our own multi-tenant logic in the plugin (e.g., a concept of “brands” within one WP site), but WordPress Multisite gives this isolation out-of-the-box.
Triggering and Scheduling Posts: To avoid slowing down the web request when an editor clicks “Publish” on an article, the plugin will offload the actual API calls to a background process:
When publish_post
fires, the plugin quickly queues a job (for example, using WP-Cron or an asynchronous task library like Action Scheduler) to handle the social posting. This returns control to WordPress immediately, so the author sees their post published without delay.
The background job, running shortly after (within seconds), fetches the post content and then iterates through each enabled social network, calling the respective API.
If all calls succeed, it can mark the post as shared (could update a meta field or log entry). If some fail, it will log errors for admins to review (and possibly schedule a retry).
This design ensures that even if posting to all 11 platforms takes, say, 10 seconds, it doesn’t make the author wait on page load. It also means if one platform is slow or down, it won’t block the others or the publishing flow.
We might implement a small queue system: e.g., a custom database table where jobs are stored (post ID, site ID, list of networks to post to) and a WP-Cron that checks every minute.
Given the scale (1000 sites, potentially many posts), a robust queue can help throttle bursts. In high-traffic scenarios, we could even dedicate a separate worker process or server for handling these API calls to not burden the web server.
Logging and Monitoring: The plugin will include a log of actions – for each post, it can list which networks it was shared to and when. If an error occurred (e.g., an expired token or API failure), the log will note it and alert the site admin (possibly via an admin notice or an email).
This feedback is important for trust in the automation. In a centralized dashboard, the Super Admin might even see aggregated logs to monitor overall system health.
Plugin Code Overview
Below is an outline of the plugin code, focusing on critical parts (initialization, settings page, and publish hook).
For brevity, we will show the structure and one example integration (Facebook). Similar code would be created for other platforms (Twitter, LinkedIn, etc.), possibly using external SDKs or raw HTTP API calls via WordPress’s HTTP API (wp_remote_post
).
central-social-publisher.php
– Main plugin file:
phpCopy<?php
/**
* Plugin Name: Central Social Publisher
* Description: Auto-publishes posts to multiple social media platforms for each brand site in a multisite network.
* Version: 1.0
* Author: [Your Name/Company]
* Network: true
*/
if (!defined('ABSPATH')) exit; // Security: exit if accessed directly
class CentralSocialPublisher {
private $options;
public function __construct() {
// Load saved options (social account tokens) for this site
$this->options = get_option('csp_options', []); // associative array of networks and creds
// Admin menu for settings
add_action('admin_menu', [$this, 'add_admin_pages']);
// Hook on post publish
add_action('publish_post', [$this, 'handle_post_publish'], 10, 2);
// (Optional) Also hook for custom post types if needed
}
public function add_admin_pages() {
add_menu_page(
'Social Publishing',
'Social Publishing',
'manage_options',
'csp-dashboard',
[$this, 'render_dashboard_page'],
'dashicons-share',
30
);
}
public function render_dashboard_page() {
if (!current_user_can('manage_options')) return;
// Handle form submission for saving credentials
if ($_POST['csp_submit']) {
check_admin_referer('csp_settings_save');
// Iterate expected fields (e.g., facebook_app_id, facebook_app_secret, facebook_page_id, facebook_token, etc.)
$new_opts = [];
// Example: Facebook
$new_opts['facebook'] = [
'page_id' => sanitize_text_field($_POST['facebook_page_id']),
'access_token' => sanitize_text_field($_POST['facebook_access_token'])
];
// (Repeat for other networks: instagram, twitter, etc., collecting their tokens/IDs from the form)
update_option('csp_options', $new_opts);
$this->options = $new_opts;
echo '<div class="updated"><p>Settings saved.</p></div>';
}
// Output the settings form
?>
<div class="wrap">
<h1>Social Publishing Settings</h1>
<form method="post" action="">
<?php wp_nonce_field('csp_settings_save'); ?>
<h2>Facebook Settings</h2>
<p>
Page ID: <input type="text" name="facebook_page_id" value="<?php echo esc_attr($this->options['facebook']['page_id'] ?? ''); ?>" />
</p>
<p>
Access Token: <input type="text" name="facebook_access_token" value="<?php echo esc_attr($this->options['facebook']['access_token'] ?? ''); ?>" />
</p>
<!-- Repeat similar sections for Instagram, Twitter, etc. Possibly provide "Connect" buttons instead of manual tokens. -->
<p><input type="submit" name="csp_submit" class="button button-primary" value="Save Settings"></p>
</form>
</div>
<?php
}
public function handle_post_publish($post_ID, $post) {
// Ensure it's a post (and not a revision), and only on first publish
if ($post->post_type !== 'post' || $post->post_status !== 'publish') return;
// Schedule background job to do the posting (using WP Cron for immediate async execution)
wp_schedule_single_event(time() + 5, 'csp_do_share', [$post_ID]);
}
// Static function to perform the actual sharing (called by cron)
public static function do_share($post_ID) {
$post = get_post($post_ID);
if (!$post) return;
// Get site-specific options
$options = get_option('csp_options', []);
$post_title = get_the_title($post);
$post_excerpt = has_excerpt($post) ? get_the_excerpt($post) : wp_trim_words($post->post_content, 50);
$post_url = get_permalink($post);
$hashtags = [];
$post_tags = wp_get_post_tags($post_ID);
foreach ($post_tags as $tag) {
$hashtags[] = '#'.preg_replace('/\s+/', '', $tag->name);
}
$hashtags_str = implode(' ', $hashtags);
$message = $post_title . " - " . $post_excerpt . " " . $hashtags_str . " " . $post_url;
// Now, for each network in options, call the respective API
if (!empty($options['facebook']['access_token'])) {
self::share_to_facebook($options['facebook'], $message, $post_ID);
}
if (!empty($options['twitter']['access_token'])) {
self::share_to_twitter($options['twitter'], $message, $post_ID);
}
// ... similarly for other networks
}
private static function share_to_facebook($fbOpts, $message, $post_ID) {
$page_id = $fbOpts['page_id'];
$token = $fbOpts['access_token'];
if (!$page_id || !$token) return;
// Prepare Facebook API call
$api_url = "https://graph.facebook.com/v16.0/$page_id/feed";
$params = [
'message' => $message,
'link' => get_permalink($post_ID),
'access_token' => $token
];
$response = wp_remote_post($api_url, ['body' => $params]);
if (is_wp_error($response)) {
error_log("Facebook share failed for post $post_ID: " . $response->get_error_message());
}
// We might handle response to check if Graph API returned an error and log accordingly.
}
private static function share_to_twitter($twOpts, $message, $post_ID) {
// Using Twitter API v2 or v1.1 - this is more complex due to OAuth 1.0a signing.
// For brevity, assume we have a Bearer token or use v2 with OAuth2.
$token = $twOpts['access_token'];
if (!$token) return;
$api_url = "https://api.twitter.com/2/tweets";
$body = json_encode(['text' => $message]);
$response = wp_remote_post($api_url, [
'headers' => [
'Content-Type' => 'application/json',
'Authorization' => "Bearer $token"
],
'body' => $body
]);
if (is_wp_error($response)) {
error_log("Twitter share failed for post $post_ID: ".$response->get_error_message());
}
// (In practice, for user-specific posting, Twitter's OAuth1.0a with user access tokens would be used.
// This example assumes a simplified approach or an OAuth2 context for brevity.)
}
}
// Initialize plugin for each site context
if (function_exists('is_multisite') && is_multisite()) {
// Only run plugin on site context, not network admin (unless providing network overview features)
if (!is_network_admin()) {
new CentralSocialPublisher();
}
} else {
// Single site usage (if not multisite)
new CentralSocialPublisher();
}
// Schedule hook needs to be registered to call do_share
add_action('csp_do_share', ['CentralSocialPublisher', 'do_share'], 10, 1);
?>
The above plugin code is a simplified representation. It shows how we attach to post publishing, schedule an async job (csp_do_share
), and how one might implement sharing for Facebook and Twitter. In a full implementation, each platform’s function (share_to_x
) would handle the specifics (including authentication).
Also, the admin settings UI in practice would be more user-friendly – likely with “Connect to Facebook” buttons that handle OAuth redirection rather than manually pasting tokens. But internally, after OAuth, we’d still store the tokens as shown.
Security considerations in code: We use check_admin_referer
for form submissions to prevent CSRF, current_user_can('manage_options')
to ensure only admins access the settings, and we sanitize inputs. The access tokens are stored in options; for stronger security, we might encrypt them before storage (using a secret key in wp-config.php
).
Additionally, when making API calls, we ensure to use HTTPS endpoints (as seen with Facebook and Twitter URLs). The plugin does not expose any data publicly – it only runs on the server side.
Performance considerations in code: The use of wp_schedule_single_event
offloads the heavy lifting. If 100 posts are published at once across the network, 100 events will be scheduled.
WordPress will sequentially execute them (WP-Cron by default runs on page loads, but we can set up a real cron or use an external cron service to handle high volume reliably).
In high scale, we might integrate a message queue or background worker system outside of WP, but the cron approach is sufficient to start.
Each API call is made via wp_remote_post
which is asynchronous from the user perspective (happening in cron). We also log errors with error_log
(which could be routed to a logging service).
For large media (images/videos), we’d ensure to not hold too much in memory at once and possibly stream if needed (especially for video uploads to YouTube/TikTok – those might be handled via their own SDKs).
Plugin Feature Summary
To recap, our Central Social Publisher plugin offers the following features to meet the requirements:
Multi-Brand Workspaces: Each site (brand) manages its own social connections and can only publish its own content. The UI is isolated per site, effectively creating separate workspaces within one central system (the multisite network). This satisfies the need for brand-specific management.
Automated, Immediate Sharing: On each post publish, all connected social accounts are updated within seconds. This ensures news is disseminated in real-time across all channels without human intervention.
Broad Social Platform Support: All requested platforms are integrated (as detailed in the table).
The plugin ensures content format is tailored to each (e.g., image vs video requirements, link handling, character limits). Including the featured image, excerpt, and hashtags in posts increases engagement on social platforms.
We leverage each platform’s API capabilities to include these elements (for example, posting images to Threads or updating Google Business with images and events).
Customization & Extensibility: The architecture allows adding more networks or customizing posting behavior. Admins can also tweak the default message templates. (Future enhancements could include scheduling posts for optimal times rather than immediate, and analytics for clicks/engagement from each post.)
Scalability: By using one plugin instance for the whole network, we reduce duplication. The database has separate tables per site, so even 1000 sites worth of social settings are just partitioned data (ensuring queries remain fast).
If needed, we can scale out the web servers or database. The design supports growth: WordPress.com itself uses a form of multisite for millions of blogs, indicating the approach can scale very far by adding hardware and caching. Our plugin’s stateless, event-driven nature means it can also be distributed (multiple WP instances processing the queue).
Security & Permissions: Only authorized users can connect accounts and publishing is triggered only by legitimate post publishes (we rely on WordPress’s authentication and roles). OAuth tokens protect user passwords. We also ensure to not expose tokens in any front-end or logs. Regular reviews of the app credentials on each platform (e.g., regenerating keys, removing unused permissions) will be advised.
With the theme and plugin in place, we next describe how to deploy this system and how a brand would start using it.
Deployment Guide
Deploying the centralized system involves setting up the WordPress Multisite and then configuring each brand’s workspace. Below is a step-by-step guide:
1. Set Up WordPress Multisite:
Install WordPress: Begin with a fresh WordPress installation on a server that can handle the expected load (Linux/Apache or Nginx, PHP 8+, MySQL/MariaDB). Ensure pretty permalinks are supported (mod_rewrite).
Enable Multisite: In wp-config.php
, add:phpCopydefine('WP_ALLOW_MULTISITE', true);
Then in the WP Admin, go to Tools → Network Setup. Choose “Sub-domains” option (since we will map top-level domains for each site). WordPress will prompt to add additional lines to wp-config.php
and to .htaccess
for multi-site configuration – do so and reload.
Network Configuration: After enabling, you become the Super Admin. Create the initial network details (network title, admin email). Now you have a Multisite network ready.
Domain Mapping: By default, additional sites might use subdomains of the main install (e.g. site1.mainnetwork.com
). We need to map each to its custom domain. You can add sites in My Sites → Network Admin → Sites → Add New, giving each a temporary address.
Then edit each site and change its “Domain” to the custom domain (e.g. brand1.com
). In modern WP (since 5.0+), domain mapping is built-in. At the DNS level, point each brand’s domain to the WordPress server’s IP.
Ensure the web server is configured to respond to those domains (wildcard or multi-host config). Set up SSL for each domain (a wildcard certificate or use Let’s Encrypt for each). After this, each brand’s site will be accessible at their own domain but powered by the central WP. (This process can be scripted to add 1000 domains, or done in batches.)
2. Install the Shared Theme:
Upload the custom theme (the “Central News Theme” we created) into /wp-content/themes/
. In Network Admin, go to Themes and Network Enable it. This makes the theme available to all sites.
For each existing site (and new one), activate the theme. You can also programmatically set this theme as the default for new sites. Each brand can now customize the theme: they will upload their logo via Customizer, set their site title/tagline, create menus for navigation, etc. (We can supply a guide to brands on how to do these basic customizations).
3. Install the Social Publisher Plugin:
Upload the “Central Social Publisher” plugin to /wp-content/plugins/
. In Network Admin, Network Activate it. This means the plugin is active on all sites automatically.
(Optionally, if some brands shouldn’t use it, we could activate per site instead, but presumably all will use it.) Upon activation, the plugin doesn’t require global config – it will simply add the “Social Publishing” menu in each site.
4. Register Social Media Apps/Keys:
For each social platform, you (or each brand) will need to register an “App” or obtain API credentials:
Facebook/Instagram: Create a Facebook App via developers.facebook.com. Configure it for Graph API, add permissions for pages manage/publish and Instagram. Generate an App ID/secret. (Our plugin could embed these globally, or each brand can have their own app – typically, one app is enough and each brand just authorizes it to manage their page.)
Twitter/X: Apply for a developer account if not already. Create a Project and an App to get API keys (and subscribe to appropriate API tier if needed for posting).
LinkedIn: Create an app on LinkedIn Developers, define the OAuth redirect URL (pointing to our site if implementing OAuth flow), and get client ID/secret.
Google (GMB & YouTube): Use Google Cloud Console to create an OAuth consent and credentials for Google My Business API and YouTube Data API.
Pinterest: Create a Pinterest developer app.
TikTok: If available, register an app via TikTok for Business developers.
Bluesky & Mastodon: These might not require registering an app (Bluesky uses app passwords, Mastodon uses user-generated tokens per instance).
Threads: No official app, possibly skip or use a placeholder.
OAuth Redirect Setup: For those using OAuth (Facebook, Google, LinkedIn, etc.), set the redirect URI to something like https://yourmaindomain.com/wp-admin/admin.php?page=csp-dashboard
(if our plugin can handle the exchange).
Our plugin code would need endpoints to catch the callback and store tokens. This is an advanced setup; to simplify, we might manually obtain long-lived tokens initially and input them. But long-term, building the OAuth flow in the plugin is ideal for user-friendliness.
This step is one-time per platform. The Super Admin (or developer) might configure the plugin with the app credentials (client IDs/secrets) possibly in a config file or a global network setting, so that individual brands don’t need to enter those, only their user tokens.
Our current plugin code sample does not show global config, but it could be extended to have a network settings page for entering global API keys (e.g., Twitter API key that is common, etc.). Each brand would then just click “Connect” and get redirected to authorize.
5. Connect Brand Social Accounts:
Now the Super Admin or individual brand admins go into each site’s Social Publishing settings (WP Admin of their site). They will see fields or buttons to connect each platform.
For example, on Brand1 site’s dashboard, the admin can connect Facebook by entering their Page ID and clicking an OAuth link to get a token (or paste a token obtained from Graph API Explorer for testing).
They would repeat for Instagram (link Instagram Business account), Twitter (authorize the app which yields a token/secret), etc., for all platforms they want to auto-post to.
Our plugin will store all these credentials in that site’s options. We advise that each brand connects only the accounts relevant to them. If a brand doesn’t have a presence on a platform (e.g., not on Bluesky or TikTok), they can ignore those – the plugin will simply not post to unconfigured networks.
We should provide documentation to the brands on how to create the tokens or authorize the app for each platform (since each has a different process). This might include step-by-step with screenshots (outside the scope of this report, but a necessary accompaniment).
6. Testing the Setup:
Before full deployment, test with a couple of sites and accounts. Publish a test post on Brand1’s site – verify that within a minute, the post appears on the connected Facebook page, Twitter feed, LinkedIn, etc. Check that the featured image and excerpt are correctly included, and hashtags appear.
Adjust any formatting issues in the plugin (for example, maybe the combined message was too long for Twitter – in which case implement truncation logic, or handle platform-specific message formatting).
Also test failure modes: e.g., revoke a token and see if the plugin logs an error on publish and doesn’t crash anything. These tests ensure reliability when scaling out.
7. Rollout to All Brands:
Once tested, proceed to add all remaining brand sites to the network (if not already done). Use automation if possible (WP-CLI or scripting) to add sites and map domains to avoid manual work for 1000 entries.
Bulk invite brand admins to their respective sites (WordPress can send user invites, or existing accounts can be added to sites). Each brand admin should get access to their site in WP.
Provide the brand teams with the Theme usage guide (how to update logo, menus, etc.) and the Social Publisher guide (how to connect their social accounts). Assist them in the initial connection process if needed, as some API credential steps can be technical.
8. Going Live and Monitoring:
As brands start publishing via the new system, monitor the server performance. Ensure object caching (e.g., Redis or Memcached) is enabled to handle many DB queries from the sites. Use a CDN for static assets to reduce load.
Monitor the WP-Cron or background tasks to ensure social posts are going out quickly. If backlog grows, consider increasing cron frequency or splitting load.
Keep an eye on API usage quotas for each platform. For example, if 1000 posts go to Twitter in a short span, ensure your Twitter app tier supports that many posts.
If not, stagger the posts or upgrade the API plan. Similar for other platforms (LinkedIn has daily post limits, etc.). Implementing a slight random delay per site’s posts can prevent a thundering herd problem at exact publish times.
Maintenance: Regularly update the theme/plugin with security patches or improvements. Because it’s centralized, updating is one action for all sites.
Back up the database often (since it holds all the content and settings). Also, periodically review connected accounts – tokens can expire (e.g., LinkedIn tokens last 60 days, Facebook tokens can expire if user changes password, etc.).
The plugin can be improved to refresh tokens automatically if the platform supports refresh tokens. For any platform API changes (they do change policies), update the integration accordingly.
By following these steps, you will deploy a scalable infrastructure where adding a new brand site or social account is straightforward. You can instantly launch new sites without separate installations (just a new site on the network), which is critical given the potential growth beyond 1000 sites.
This centralized approach means minimal overhead per additional site – the code and server are shared, so it’s mostly a matter of content and configuration.
Using the Platform for Social Publishing
Once deployed, here’s how a typical brand user (editor/admin of a news site) would use the platform day-to-day:
Publish Content as Usual: Journalists or editors create articles on the WordPress site just as they normally would – writing the post, setting a featured image, adding tags/categories, and clicking Publish.
No extra steps are needed to share on social media (though optional customization fields can be provided, e.g., a custom tweet text field if desired).
Automatic Social Sharing: Within moments of publishing, the editor can trust that the article has been announced on all the configured social channels.
For example, the editor publishes an article “Breaking News X”. The plugin immediately posts a Facebook update on the brand’s page with the article link and image, tweets a summary with hashtags on the brand’s Twitter account, posts the image and excerpt on Instagram, and so on for LinkedIn, Threads, etc.
This saves the social media manager’s time – they no longer need to manually copy-paste links after publishing.
Verification: The brand’s social media manager might verify the posts went out (this can be done by simply checking their social feeds).
Additionally, the plugin’s log (accessible in WP Admin) will show a record like “Article ID 123 shared to: Facebook ✅, Twitter ✅, LinkedIn ✅, Instagram ✅ …” or show any errors.
This transparency helps in case a token expired – the manager would see, for example, “Twitter ❌ (invalid credentials)” and know to re-connect the Twitter account.
Managing Accounts: If the brand rebrands or changes social accounts (say they create a new Facebook Page or add a new platform like they join Bluesky), they can go into the Social Publishing settings and update the credentials. It’s all centralized in one screen per site.
There’s no need to fiddle with multiple plugins or external services – one dashboard controls it all. Conversely, if they decide to stop posting to a platform, they can simply disconnect it.
Content Optimization: Over time, users can refine how content is shared. For instance, they might observe that including too many hashtags on LinkedIn is not effective, so they reduce those in the template.
Or they might start using the plugin’s ability to define a custom message for Twitter vs. Facebook to better suit each audience. Our plugin’s UI can evolve to accommodate these needs (perhaps a meta box in the post editor to override the default message per platform for that post, etc., which advanced users can use).
Analytics (Future Scope): While not specified, it’s worth noting that the effectiveness of auto-posting can be tracked. Each social platform provides analytics.
An enhancement could be the plugin retrieving some data (likes, shares, clicks) via APIs and showing it in the dashboard, so brands can see the impact. This isn’t in the initial requirement, but the architecture is ready for such extensions.
Throughout usage, performance remains a priority. The theme delivers pages to readers quickly, and the plugin operates mostly in the background.
Even as more sites and posts are added, the system leverages WordPress Multisite’s proven scalability (separating data per site and allowing scaling horizontally).
If the load grows (e.g., heavy traffic or extremely frequent posting), we can scale by upgrading the server or splitting database reads across replicas. The design already includes caching and non-blocking operations to handle high volume.
Security maintenance: We instruct all brand admins to keep their account tokens secure – e.g., not share their WP admin login or those tokens with unauthorized persons.
Our system uses least-privilege tokens (only post permissions), which mitigates risk. We will also keep WordPress and the plugin up to date to patch any security issues that arise.
Conclusion
In summary, the proposed solution provides a unified platform for managing 1000+ news websites and their social media presence at scale. By using WordPress Multisite, we achieve central management of code and configuration while isolating each brand’s content and settings.
The custom theme ensures a consistent, high-performance website for each brand, and is easy to maintain centrally. The Social Publisher plugin fulfills the need for instant, automated social media distribution – turning each WordPress site into a content hub that syndicates news across all major networks with minimal human effort.
We prioritized performance (through caching, async processing, and scalable architecture) and security (through role-based access, secure token storage, and OAuth protocols).
This system is built from scratch to fit the organization’s needs, avoiding the limitations of off-the-shelf plugins and allowing full control over data and functionality. It can be further customized as needs evolve (for example, adding new social networks or advanced scheduling features).
By implementing this system, the organization can significantly streamline its digital operations: editors focus on content, and the platform takes care of multi-channel publishing.
This not only saves time (sharing to a dozen platforms happens in one click), but also ensures wider and faster reach for news articles, thereby increasing audience engagement across the board.
With the provided code and deployment guide, the IT team can proceed to build and launch this platform, confident that it will meet the demands of a large-scale, modern news network.